VB.NET Looping Statements: Syntax and Examples [VB.NET Tutorials]
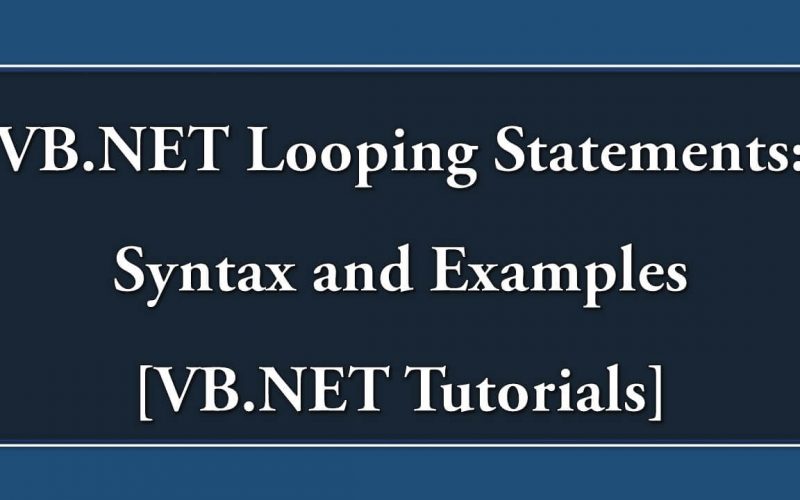
Loop statements in programs are used to execute a block of program codes multiple times. Loop statements allow us to run a set of program codes which are written inside the loop for multiple times. The codes written inside the loop block are executed while only the given condition is true and the loop automatically ends when the condition false. VB.Net supports several types of loop statements and loop control statements.
VB.Net supports the following Loop statements:
- Do Loop
- For Next
- For Each Next
- While End While
- With End With
VB.Net also supports some controls statements. Typically the statements inside the loop will execute one by one; control statements change execution from this typical sequence.
The control statements in VB.Net are given below:
- Exit Statement
- Continue Statement
- GoTo Statement
Now let me describe all of the loop statements with examples.
1. Do Loop
The Do Loop repeats the group of statements while or until the given boolean condition is true. The Do Loop statements are terminated by the Exit Do statement.
There are two methods of Do Loop. The first method is entry loop and the second method is exit do loop. In entry do loop the boolean condition is checks first, and the exit Do-loop checks the boolean condition after the execution of loop statements. The syntax of Do Loop is given below.
Method 1
Do {While | Until} condition [statement 1] [continue Do] [statement 2] [Exit Do] [statement X] Loop
Method 2
Do [statements 1] [Continue Do] [statements 2] [Exit Do] [Statements X] Loop {While | Until} condition
Now let us see an example program using Do Loop which finds the sum of the first n positive integers.
EXAMPLE PROGRAM (METHOD 1)
Module Module1 Sub Main() 'Declare two variables Dim a As Integer = 1 Dim sum As Integer = 0 'do loop Do sum = sum + a a = a + 1 Loop While (a < 10) 'Print the sum Console.WriteLine(sum) Console.ReadLine() End Sub End Module
The output of the above program will be 55.
EXAMPLE PROGRAM (METHOD 2)
Module Module1 Sub Main() ' Declaring Variables Dim a As Integer = 1 Dim sum As Integer = 0 'Execution of Do Loop Do sum = sum + a a = a + 1 Loop Until (a = 10) Console.WriteLine(sum) Console.ReadLine() End Sub End Module
The output of the above program will be 55.
2. For…Next Loop
The for loops repeats a block of statements for a specified number of times. Here the loop also counts the number of the loop executions during every loop execution.
The syntax and example program of the For…Next Loop is given below.
SYNTAX
For counter [ Datatype ] = min_value To max_value [ Step ] [ statement 1 ] [ Continue For ] [ statement 2 ] [ Exit For ] [ statement X ] Next [ count ]
Now let’s see how this loop construct works using the below example program. This program will calculate and print the first n positive integers.
EXAMPLE PROGRAM
Module Module1 Sub Main() 'Declare variables Dim a As Integer Dim sum As Integer 'Execution of for loop For a = 0 To 10 Step 1 sum = sum + a Next Console.WriteLine(sum) Console.ReadLine() End Sub End Module
The above program will output the sum of first ten positive integers 55.
3. For Each…Next Loop
The For Each…Next is an another looping statement which repeats the block of statements for every element in the given group of items. This Loop statement is used in VB.Net for the manipulation of an array of elements.
The syntax and the example program of this loop are given below.
SYNTAX
For Each element [ Datatype ] In group_of_elements [ statement 1 ] [ Continue For ] [ statement 2 ] [ Exit For ] [ statement X ] Next [ element ]
Now let us deal with an example program for this loop.
EXAMPLE PROGRAM
Module Module1 Sub Main() 'Declaring the variables Dim MyArray() As Integer = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10} Dim element As Integer 'To display the array items For Each element In MyArray Console.WriteLine(element) Next Console.ReadLine() End Sub End Module
The output of the above program is as shown below.
1 2 3 4 5 6 7 8 9 10
4. While… End While Loop
The While… End While loop executes the set of following statements while the given condition is true. The statements of while loop are enclosed within While and End While statements.
The syntax and the example program of the While loop construct are given below.
SYNTAX
While condition [ statement 1 ] [ Continue While ] [ statement 2 ] [ Exit While ] [ statement X ] End While
Example program to print the first ten positive integers.
EXAMPLE PROGRAM
Module Module1 Sub Main() 'Variable declaration Dim a As Integer = 1 'Execution of while loop While a <= 10 Console.WriteLine(a) a = a + 1 End While Console.ReadLine() End Sub End Module
The output of the above program is given below.
1 2 3 4 5 6 7 8 9 10
5. With… End With
The With… End With is not a looping statement, but it also counts as a looping statement. This is called a loop statement because it executes a series statements multiple times by referring to a single object or structure.
The syntax and example program of With… End With is given below.
SYNTAX
With object [ statements ] End With
EXAMPLE PROGRAM
Module Module1 Public Class Student Public Property Name As String Public Property Address As String Public Property Age As Integer Public Property Grade As String End Class Sub Main() Dim Student1 As New Student With Student1 .Name = "Adeeb ACP" .Address = "Southampton Drive Winchester, AD 12345" .Age = 15 .Grade = "A" End With With Student1 Console.WriteLine(.Name) Console.WriteLine(.Address) Console.WriteLine(.Age) Console.WriteLine(.Grade) End With Console.ReadLine() End Sub End Module
The output of the above program code shows below.
Adeeb ACP Southampton Drive Winchester, AD 12345 15 A
NESTING OF LOOPS
The using of one loop statement in another loop statement is known as Nesting of Loops. The nested loop statements allow us to create more complex programs.
SYNTAX FOR NESTED LOOPS:
The three loop statements support in VB.Net are nested For Loop, nested While Loop and nested Do…While Loop.
Nested For Loop:
For loop_counter1 [ Datatype 1 ] = start_value1 To end_value1 [ Step step1 ] For counter2 [ As datatype2 ] = start_value2 To end_value2 [ Step step2 ] ... Next [ Loop_counter2 ] Next [ loop_counter 1]
Nested While Loop:
While first_condition While second_condition ... End While End While
Nested Do…While Loop:
Do { While | Until } first_condition Do { While | Until } second_condition ... Loop Loop