C Plus Plus Functions [C++ Tutorials-5]
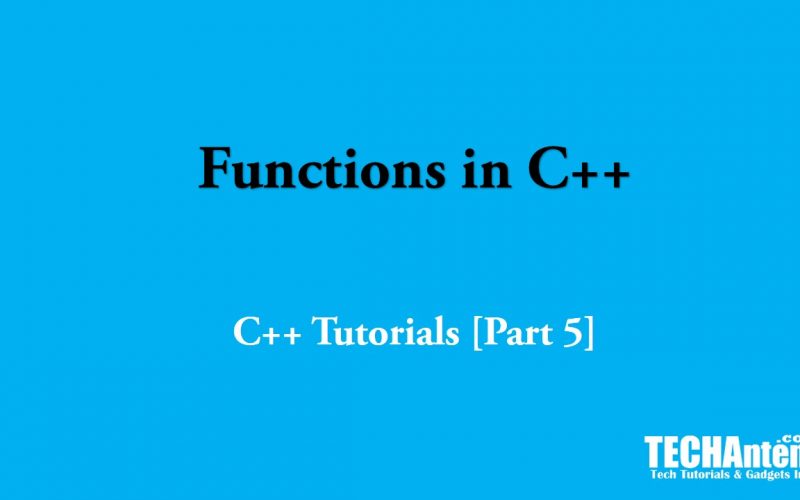
C Plus Plus Functions
C Plus Plus Functions are the named set of statements that can be processed independently. It helps to reduce the complexity of a program. It is difficult to implement a large program even its algorithm is written. To implement such large programs with a case, it should be split into a number of independent tasks, which can be easily designed, implemented and managed.
This process of splitting a large program into small manageable tasks and designing them independently is known as modular programming.
A repeated group of instructions in a program can be organized as a function.
Advantages of C Plus Plus Functions
- Modular programming
- Reduction in the amount of work and development time
- Program and function debugging are easier
- Division of work is simplified
- Reduction in size of the program due to code reusability
- Library of functions can be implemented by combining well designed and tested functions.
Functions in C++ are classified into two. They are described below.
Built-in Functions:
C++ has a wide range of library functions for diverse applications. The function prototype for these are defined in the different header files.
Eg: The header file conio.h contains clrscr(), getch() functions.
User defined functions:
The user defined functions are defined by the programmer for special purposes in a program.
Function Prototype in C++ (Function decelaration)
All C Plus Plus functions must be declared before they are use in a program. C++ allows the declaration of functions in the calling program with the help of a function prototype.
The general form of a function prototype in the calling program is given below.
type function_name (argument type);
Eg:
int total (int, int);
As you can see from the above example and syntax, the function declaration provides the following information to the compiler.
- The type of the value returned fom the function.
- The name of the function, and
- The number and the types of the arguments that are passed to the function.
Defining a function in C++
The defining of C Plus Plus functions contains two parts: the function header and function body.
The function header contains function name, function return type and parameter list.
The function body contains the statements of the function.
Example:
int large (int a, int b) { if (a>b) return a; else return b; }
This function will return a if a>b else returns b.
Calling function in C++
A function can be called or invoked from another function by using it’s name. The function name must be followed by a set of actual parameters seperated by commas.
Example:
#include<iostream.h> #include<conio.h> int large(int, int); //funtion prototype void main( ) { int a, b; clrscr( ); cout<<"Enter two numbers: "; cin>>a>>b; c= large(a, b); //function call cout<<"Large numbers: "<<c; } int large (int x, int y) //function definition { int z; if (x>y) return x; else return y; }
The above program finds the large number from two given numbers.
Actual and Formal Parameters
The parameters specified in the function call are known as actual parameters and those specified in the function definition are known as formal parameters. For instance, in the above program the variables ‘a’ and ‘b’ are called actual parameters and the variables ‘x’ and ‘y’ are called formal parameters.
Global and Local Variables
A variable declared within the body of a function will be available only within the function. Such variables are called local variables.
A variable that is declared outside any function is called a global variable. These variables are accessible to all functions which follow their declarations. A variable declared at the top, before any function is defined,will be available to all function.
Example Program #include<iosream.h> #include<conio.h> float area; //global variable float circle (int); void main( ) { int r; clrscr( ); cout<<"Enter the circle radius: "; cin>>r; area = circle (r); cout<<"Area : "<<area; } float circle(int r) { float p; //local variable p = 2*22.0 / 7*r ; area = 22.0/ 7*r*r; cout<<"Perimeter : "<<p; return area; }
Here the variable ‘area’ is a global variable and ‘p’ is a local variable. Here ‘p’ is only available in the function circle( ).
We will discuss more about the C plus plus functions in following posts.