C Program to Check a Armstrong Number with Algorithm
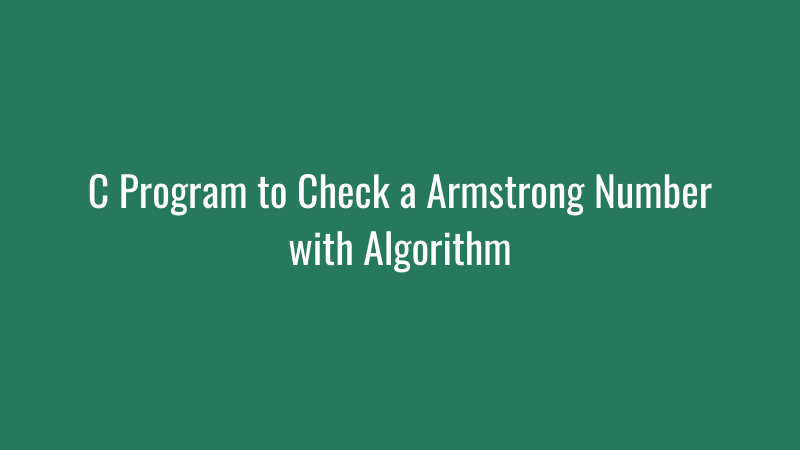
Here we discuss the C Program to Check a Armstrong Number with the help of step by step algorithm.
Aim:
Write a C Program to Check weather a given number is Armstrong or not.
Algorithm:
Step 1: Begin
Step 2: Read an integer value and assign to a variable num
Step 3: Copy the value of num into a variable temp
Step 4: Initialize an integer variable result as 0 and repeat steps 5 to 7 till the condition(temp!=0) become false
Step 5: Calculate reminder variable,r using formula : r= temp%10
Step 6: Update result as result+(r*r*r)
Step 7: Update temp as temp/10
Step 8: Check whether num==result, if yes, do step 9 else step 10
Step 9: Print ” Given number is an Armstrong ”
Step 10: Print ” Given number is not an Armstrong ”
Step 11: End
C Program to Check a Armstrong Number
//include header files
#include
#include
void main()
{
// variable's declarations and initialization
int num, result=0, r, temp;
clrscr();
//read a number > 0
printf("Enter a number greater than zero :");
scanf("%d", &num);
//while loop to check whether a given number is Armstrong or not
while( temp!= 0 )
{
r= temp % 10;
result= result + ( r*r*r);
temp= temp / 10;
}
//print result
if( temp == result )
printf("\n %d is an Armstrong number. ");
else
printf("\n %d is not an Armstrong number. ");
getch();
}
OUTPUT
Enter a number greater than zero: 371
371 is an Armstrong number.
Enter a number greater than zero:300
300 is not an Armstrong number.